NULL vs FALSE vs Empty String vs Zero vs NaN: Key Differences in JavaScript
JavaScript
November 30, 2023
A Comprehensive Guide to Handling NULL, FALSE, Empty Strings, Zero, & NaN in JavaScript
This article provides a comprehensive overview of commonly misunderstood values in JavaScript, equipping you with practical knowledge for managing them effectively in your code.
Introduction
JavaScript developers often encounter various "falsy" values like NULL
, FALSE
, empty strings (""
), zero (0
), and NaN
. These values may look similar, but each has unique properties and uses, impacting how your code behaves. Misunderstanding these differences can lead to unexpected bugs and challenges in handling conditional logic, data validation, and more. In this guide, we'll break down the differences between NULL
, FALSE
, empty strings, zero, and NaN
, and when to use each in JavaScript.
Table of Contents
- Overview of Falsy Values in JavaScript
- Understanding NULL
- FALSE in JavaScript
- Empty String: ""
- Zero: 0
- NaN (Not-a-Number)
- NULL vs FALSE vs Empty String vs Zero vs NaN: Key Differences
- Best Practices for Working with Falsy Values
- Conclusion
1. Overview of Falsy Values in JavaScript
In JavaScript, a "falsy" value is a value that evaluates to false
when used in a Boolean context. JavaScript's loosely typed nature allows different data types to be coerced to true
or false
. The primary falsy values include:
false
0
""
(empty string)null
undefined
NaN
Each of these values behaves differently depending on the context in which they're used.
2. Understanding NULL
NULL
in JavaScript represents the intentional absence of a value. It is often used to indicate that a variable or object property has no value.
- Type:
object
- Value:
null
(explicitly assigned) - Use case: Use
NULL
when you want to explicitly declare a variable as "empty" or "unassigned."
Example:
let user = null; // User has no value
3. FALSE
in JavaScript
FALSE
is a Boolean value indicating "not true." In JavaScript, it's often used as a flag or in conditional statements to denote false conditions.
- Type:
boolean
- Value:
false
- Use case: Use
FALSE
when you want to check a Boolean condition or represent something as false.
Example:
let isLoggedIn = false; // User is not logged in
4. Empty String: ""
An empty string (""
) is simply a string with no characters. It's useful for clearing input fields or when text is optional.
- Type:
string
- Value:
""
- Use case: Use an empty string when you want to initialize a variable as a string with no characters.
Example:
let username = ""; // Username has no text
5. Zero: 0
0
is the numeric representation of "nothing" in JavaScript. It is often used in counters, array indices, and numeric calculations.
- Type:
number
- Value:
0
- Use case: Use zero when a number with no value is needed, like initializing a counter.
Example:
let score = 0; // Initial score is zero
6. NaN
(Not-a-Number)
NaN
stands for "Not-a-Number" and is used when a calculation or operation yields a non-numeric result.
- Type:
number
- Value:
NaN
- Use case:
NaN
is often used in error handling to detect if a calculation failed.
Example:
let result = parseInt("Hello"); // NaN, as "Hello" is not a number
7. NULL vs FALSE vs Empty String vs Zero vs NaN: Key Differences
Value | Type | Description | Use Case |
---|---|---|---|
null | object | Explicitly means no value | Use to represent empty values |
false | boolean | Boolean false | Use in conditional checks |
"" (empty string) | string | String with no characters | Use when text input is empty |
0 | number | Represents no numerical value | Use in counters or calculations |
NaN | number | Represents an invalid number | Use in calculations or error handling |
Common Differences:
- Conditional Statements: All of these values evaluate as
false
in conditional statements, so it's important to check for each explicitly when needed. - Type Checking: Each falsy value has a different type, so type-checking can prevent misinterpretations in code.
- Assignments:
null
is assigned intentionally, whileNaN
results from calculations or conversions.
8. Best Practices for Working with Falsy Values
When working with NULL
, FALSE
, empty strings, zero, and NaN
, follow these best practices to maintain clean and bug-free code:
- Use Type Checking: Use
typeof
orinstanceof
to ensure you're working with the expected type.
if (typeof variable === "string") {
// Do something with string
}
- Use Strict Comparison: Use
===
instead of==
to avoid type coercion, which can lead to unexpected behavior.
if (variable === null) {
// Do something if variable is null
}
- Explicitly Check for NaN: Use
isNaN()
to check for NaN values sinceNaN !== NaN
.
if (isNaN(value)) {
// Handle NaN cases
}
- Initialize Variables: Set initial values for variables, using
null
or an empty string if necessary, to avoidundefined
. - Handle False Cases Separately: Remember that
0
, empty strings,null
,NaN
, andfalse
all evaluate asfalse
in conditionals. Be explicit in your checks to avoid confusion.
9. Conclusion
Understanding the differences between NULL
, FALSE
, empty strings, zero, and NaN
is essential for writing clean, error-free JavaScript code. Each of these values has a specific purpose and meaning. By knowing when to use each, and implementing best practices, you can write more predictable and reliable code. Keep this guide as a reference when working with JavaScript's falsy values, and you'll be able to handle different data types with confidence.
Is this article helpful?
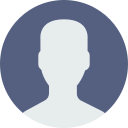
Admin
About Author
A full stack web developer specializing in frontend and backend web technologies. With a wealth of experience in building dynamic and robust web applications, he brings expertise and insights to his articles, providing valuable guidance and best practices for fellow developers. Stay tuned for more useful content.
Share the good stuff on social media and earn appreciation.