3 Ways to Check if a Function is Defined in JavaScript
JavaScript
November 14, 2023
How to Check if a Function is Defined in JavaScript?
When developing JavaScript applications, there may be cases where you need to check if a specific function is defined before calling it. This can help prevent runtime errors that occur when you try to invoke a function that hasn’t been defined yet.
In this blog post, we’ll explore the different ways to check if a function is defined in JavaScript and how to handle undefined functions gracefully.
Table of Contents
- Checking if a function is defined using
typeof
- Checking with
window
orglobalThis
object - Handling undefined functions with
try...catch
- Example Scenarios
1. Checking if a Function is Defined Using typeof
One of the most straightforward and reliable ways to check if a function is defined is by using the typeof
operator. In JavaScript, the typeof
operator returns a string indicating the type of the operand. If the function is defined, typeof
will return "function"
. If it’s not defined, it will return "undefined"
.
Here’s a simple example:
if (typeof myFunction === "function") {
// Function is defined, we can safely call it
myFunction();
} else {
console.log("myFunction is not defined.");
}
Explanation:
typeof myFunction
checks the type ofmyFunction
.- If the result is
"function"
, it indicates thatmyFunction
is defined. - Otherwise,
"undefined"
is returned, meaning that the function doesn't exist.
When to Use typeof
This approach is particularly useful when you are dealing with optional or dynamically loaded JavaScript code. It ensures that you won't encounter a runtime error by trying to invoke an undefined function.
2. Checking with window
or globalThis
Object
In a browser environment, functions defined in the global scope are properties of the window
object. In a Node.js or more modern JavaScript environments, you can use globalThis
to access the global object. You can check if a function exists as a property of these objects.
Example using the window
object (for browsers):
if (typeof window.myFunction === "function") {
// myFunction is defined
myFunction();
} else {
console.log("myFunction is not defined.");
}
Example using globalThis
(for universal JavaScript):
if (typeof globalThis.myFunction === "function") {
// myFunction is defined
globalThis.myFunction();
} else {
console.log("myFunction is not defined.");
}
Explanation:
window.myFunction
orglobalThis.myFunction
will reference the function if it’s globally defined.- The same
typeof
check is applied to ensure it’s a function before calling it.
When to Use window
or globalThis
This method is useful when you want to be explicit about the scope and make sure you are checking the global environment for the function.
3. Handling Undefined Functions with try...catch
Another way to handle potentially undefined functions is to use a try...catch
block. This method allows you to safely attempt to call a function and catch any errors if the function is not defined.
try {
myFunction(); // Try to call the function
} catch (e) {
console.log("myFunction is not defined or cannot be executed.", e.message);
}
Explanation:
try
attempts to invoke the function.- If the function is not defined, the code inside
catch
is executed, preventing the script from crashing. - The error message can be logged or handled accordingly.
When to Use try...catch
This method is helpful when you want to handle not only undefined functions but also any other potential runtime errors that might occur when calling a function.
4. Example Scenarios
Example 1: Checking for a Plugin Function
Imagine you’re building a website that optionally uses a plugin. You may want to check if a plugin-specific function is available before trying to use it.
if (typeof jQuery.fn.myPlugin === "function") {
// The plugin is available, proceed to use it
$("div").myPlugin();
} else {
console.log("myPlugin is not available.");
}
Example 2: Dynamically Loaded Functions
In a single-page application (SPA), you might load different scripts dynamically. Before calling a function from a script that may or may not have loaded, you can perform a check like this:
function callDynamicFunction() {
if (typeof dynamicFunction === "function") {
dynamicFunction();
} else {
console.log("dynamicFunction is not yet loaded.");
}
}
Example 3: Fallback Logic
Sometimes, when a function is not defined, you might want to provide fallback logic or call an alternative function.
if (typeof customLogFunction === "function") {
customLogFunction("This is a custom log message.");
} else {
console.log("This is a fallback log message.");
}
Conclusion
In JavaScript, there are several ways to check if a function is defined:
- The
typeof
operator is the simplest and most widely used approach. - You can also check the
window
orglobalThis
object to look for global functions. - For more robust error handling, using
try...catch
ensures your application doesn’t break when calling undefined functions.
By incorporating these techniques, you can build more reliable and error-tolerant JavaScript applications. Always ensure that your checks are in place, especially in scenarios involving dynamic code or optional libraries.
Is this article helpful?
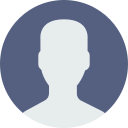
Admin
About Author
A full stack web developer specializing in frontend and backend web technologies. With a wealth of experience in building dynamic and robust web applications, he brings expertise and insights to his articles, providing valuable guidance and best practices for fellow developers. Stay tuned for more useful content.
Share the good stuff on social media and earn appreciation.