10 Common JavaScript Mistakes & How to Avoid Them
JavaScript
November 29, 2023
Top JavaScript Mistakes — How to Spot and Fix Them
JavaScript is one of the most popular programming languages, used extensively for web development. While it's powerful and flexible, it can be easy to make mistakes—especially for beginners. Even experienced developers sometimes fall into common pitfalls that lead to bugs or unexpected behavior. In this article, we'll explore 10 of the most common JavaScript mistakes and show how to avoid them.
1. Misunderstanding Variable Scope
The Mistake: JavaScript has both function scope and block scope. Variables declared with var
are function-scoped, while those declared with let
and const
are block-scoped. A common mistake is assuming that variables declared inside a block are not accessible outside of it, so they may conflict with variables with same name in other code block.
Example:
if (true) {
var x = 10;
}
console.log(x); // 10 (Function-scoped)
How to Avoid: Use let
or const
instead of var
to ensure variables are block-scoped and only accessible within the appropriate block.
if (true) {
let x = 10;
}
console.log(x); // ReferenceError: x is not defined
2. Incorrect Usage of this
The Mistake: The value of this
can change depending on how a function is called. Many developers mistakenly expect this
to always refer to the current object, but it depends on the execution context.
Example:
const obj = {
name: 'John',
sayName: function() {
console.log(this.name); // Correctly refers to 'John'
setTimeout(function() {
console.log(this.name); // Incorrectly refers to the global object
}, 1000);
}
};
obj.sayName();
How to Avoid: Use arrow functions for callbacks, as they inherit this from the enclosing context.
setTimeout(() => {
console.log(this.name); // Correctly refers to 'John'
}, 1000);
3. Failing to Declare Variables
The Mistake: If you assign a value to a variable without declaring it with var
, let
, or const
, JavaScript automatically creates a global variable. This can cause unintended side effects and bugs.
Example:
function example() {
undeclaredVar = 10; // Global variable
}
How to Avoid: Always declare variables with let
, const
, or var
.
function example() {
let declaredVar = 10; // Block-scoped variable
}
4. Not Using Strict Equality (===
)
The Mistake: JavaScript's ==
performs type coercion, which can lead to unexpected results when comparing values of different types.
Example:
console.log(0 == false); // true
console.log('' == false); // true
How to Avoid: Always use ===
for comparisons, which checks both value and type.
console.log(0 === false); // false
console.log('' === false); // false
5. Modifying Objects or Arrays Declared with const
The Mistake: Developers often assume that declaring an object or array with const
makes it immutable, but const
only prevents reassignment. The contents of the object or array can still be changed.
Example:
const arr = [1, 2, 3];
arr.push(4); // This is allowed, `arr` is still mutable
console.log(arr); // [1, 2, 3, 4]
How to Avoid: If you want to make an object immutable, use libraries like Object.freeze()
.
const obj = Object.freeze({ name: 'John' });
obj.name = 'Jane'; // Fails silently in non-strict mode or throws an error in strict mode
6. Misusing Floating Point Numbers
The Mistake: JavaScript uses floating-point arithmetic, which can lead to unexpected results when performing simple calculations like addition or subtraction.
Example:
console.log(0.1 + 0.2); // 0.30000000000000004
How to Avoid: Use a technique like rounding or the toFixed()
method to handle floating-point arithmetic more accurately.
console.log((0.1 + 0.2).toFixed(1)); // 0.3
7. Ignoring Asynchronous Code Execution
The Mistake: JavaScript is asynchronous, and functions like setTimeout
, fetch
, and promises
do not execute immediately. Ignoring this can lead to bugs when code executes out of order.
Example:
console.log('Start');
setTimeout(() => console.log('Timeout'), 0);
console.log('End');
// Output: 'Start', 'End', 'Timeout'
How to Avoid: Use async/await
or promises to handle asynchronous code properly.
async function example() {
console.log('Start');
await new Promise(resolve => setTimeout(resolve, 1000));
console.log('End');
}
example();
8. Incorrectly Using for
Loops with Asynchronous Code
The Mistake: Using for
loops to iterate over asynchronous operations can cause all iterations to run at the same time, leading to unpredictable behavior.
Example:
for (var i = 0; i < 5; i++) {
setTimeout(() => console.log(i), 1000); // Prints 5 five times
}
How to Avoid: Use let
for block-scoped iteration or async/await
with promises.
for (let i = 0; i < 5; i++) {
setTimeout(() => console.log(i), 1000); // Prints 0, 1, 2, 3, 4
}
9. Forgetting to Handle Errors in Promises
The Mistake: Promises allow for asynchronous code execution, but forgetting to handle errors can leave your code vulnerable to failures that are difficult to debug.
Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
How to Avoid: Always include a .catch()
block or use try/catch
with async/await
to handle potential errors in promises.
async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
10. Confusing Function Declarations & Expressions
The Mistake: Developers often confuse function declarations with function expressions. Declarations are hoisted, meaning they can be called before their definition. Expressions, however, are not hoisted.
Example:
sayHello(); // Works because function declaration is hoisted
function sayHello() {
console.log('Hello!');
}
How to Avoid: Be mindful of the difference between declarations and expressions, and use the appropriate one for your needs.
const sayHello = function() {
console.log('Hello!');
};
sayHello(); // Must be called after the expression
Conclusion
JavaScript's flexibility makes it a versatile and powerful language, but it also comes with its share of potential pitfalls. By understanding and avoiding these common mistakes, you can write cleaner, more reliable code. Whether you're a beginner or an experienced developer, keeping these tips in mind will help you avoid frustrating bugs and improve your JavaScript programming skills.
Also read: Var Vs Let Vs Const in JavaScript
Is this article helpful?
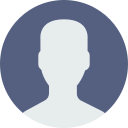
Admin
About Author
A full stack web developer specializing in frontend and backend web technologies. With a wealth of experience in building dynamic and robust web applications, he brings expertise and insights to his articles, providing valuable guidance and best practices for fellow developers. Stay tuned for more useful content.
Share the good stuff on social media and earn appreciation.